Table of Contents
Introduction Steps for making a basic calculator in Python Prompting the user for input taking input for two variables from user To evaluate each function, define and add operators or functions Apply conditional statements (if...elif...else branching) ConclusionIntroduction
Practice is essential to learning any tool that supports the IT sector. There are no quick cuts when it comes to implementing modern programming languages like Python.
When we set aside time to concentrate on simple Python project ideas, mastering this widely used programming language is a goal we can achieve.
Let’s learn a little bit more about Python before we look at some great Python applications.
Python programming is the best option for a wide range of projects, from easy operating systems to basic web apps, thanks to its simplicity and versatility.
Its simple syntax encourages readability, which considerably lowers the cost of software maintenance.
Python allows programmers to think of code as both data and functionality since it is both object-oriented and functional.
Additionally, it works with any operating system, enabling users to create native programs for both Windows and Mac computers.
Building calculators is a good first project for new Python developers. Our ability to construct a basic program, such as a calculator, will improve as you work to fully comprehend the language’s basics.
This blog will show you how to build a basic calculator that, based on the user’s input, may add, subtract, multiply, or divide.
The following Python programming concepts should be known to you in order to understand this example:
- Python Functions
- Python Function Arguments
- Python User-defined Functions
We may use if-elif
statements to develop a basic calculator and an advanced calculator as well.
Python programming is a simple and effective technique since it allows for evaluations and variable manipulation to make it function as needed.
This blog helps us understand a simple Python command-line calculator application where we will explore Python’s arithmetic operators, functions, and conditional expressions. and will develop our calculator program in Python by learning how to handle user input.
The process of writing the calculator application in Python will be broken down into a series of easy phases.
Making a simple calculator application in Python that can do operations like addition, subtraction, multiplication, and division—all of which depend on the input provided by the user—will aid in better understanding the concepts.
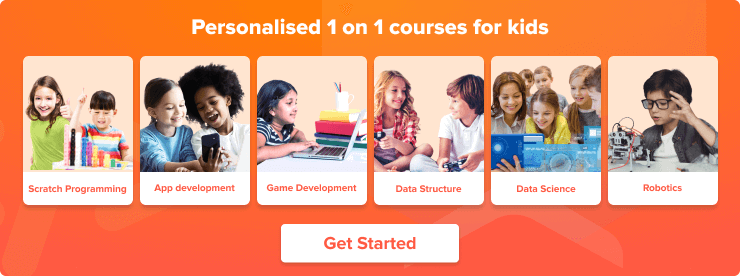
Steps for making a basic calculator in Python
- Prompting the user for input. In other words, we will take input for two variables from user.
- To evaluate each function, define and add operators or functions like
add()
,subtract()
,multiply()
, anddivide()
. - Apply conditional statements (
if...elif...else
branching) to make it function how the user chooses in order to make it comparable to the calculator.
Young programmers may enhance their general coding skills by learning about ideas like conditionals, while loops, and more. Additionally, it offers command-line programming, math, and number training.
This kind of coding project brings up a wide range of opportunities. A prospective coder may get a jump start on several exciting jobs and interests of the future, including video games, mobile apps, and artificial intelligence!
Let’s get started and learn by performing each step in the process of writing a Python calculator program.
Step 1: Prompting the user for input taking input for two variables from user
Using Python’s input()
method, we will collect user input in this step. To carry out any mathematical operations, it works exactly as when we enter numbers into a traditional calculator.
We’ll ask the user to enter two variables, using the input()
method for each variable.
num_1 = input('Please, Enter the first number: ')
num_2 = input('Please, Enter the second number: ')
These input numbers are stored in variables num1 and num2.
Please, Enter the first number: 10
Please, Enter the second number: 20
Step 2: To evaluate each function, define and add operators or functions like add(), subtract(), multiply(), and divide()
In order to develop the calculations for the calculator program in Python, we are now creating functions to carry out mathematical operations, including addition, subtraction, multiplication, and division.
In order to encourage the user to do mathematical operations on integers rather than strings, we also updated our input functions to accept integers.
num_1 = int(input('Please, Enter the first number: '))
num_2 = int(input('Please, Enter the second number: '))
# arithmetic operation: Addition
print('Addition of {} + {} = '.format(num_1, num_2))
print(num_1 + num_2)
# arithmetic operation: Subtraction
print(' Subtraction of {} - {} = '.format(num_1, num_2))
print(num_1 - num_2)
# arithmetic operation: Multiplication
print('Multiplication of {} * {} = '.format(num_1, num_2))
print(num_1 * num_2)
# arithmetic operation: Division
print('Division of {} / {} = '.format(num_1, num_2))
print(num_1 / num_2)
Please, Enter the first number: 10
Please, Enter the second number: 20
Addition = 10 + 20 =
30
Subtraction = 10 - 20 =
-10
Multiplication = 10 * 20 =
200
Division = 10 / 20 =
0.5
Each of the four fundamental arithmetic operations in Python has been discussed above using the format() function.
The format() methods fill in the blanks and format the output. Now, the user’s input has been calculated for each of the stated arithmetic operations.
We must make it operate in accordance with the user’s preference because all functions are being carried out as outlined for the two numbers.
We’ll employ conditional statements—if...elif...else
branching—so that the program only does the operations the user selects, much like a real calculator.
Step 3: Apply conditional statements (if…elif…else branching) to make it function how the user chooses in order to make it comparable to the calculator
We will define each of the arithmetic operations as a function using the def function in Python in order to make it user-based.
The user’s input for the mathematical operations they want to carry out will once again be asked for.
# Function to perform the arithmetic operation: Addition
def add(num_1, num_2):
return num_1 + num_2
# Function to perform the arithmetic operation: Subtraction
def subtract(num_1, num_2):
return num_1 - num_2
# Function to perform the arithmetic operation: Multiplication
def multiply(num_1, num_2):
return num_1 * num_2
# Function to perform the arithmetic operation: Division
def divide(num_1, num_2):
return num_1 / num_2
print("Hi, This is the Basic console based calculator!\n")
while True:
print("Please select which of the following arithmetic operation you want me to perform-\n\n" \
"1. Add\n" \
"2. Subtract\n" \
"3. Multiply\n" \
"4. Divide\n" \
"5. Exit\n")
# Taking the input from the user for which arithmetic operation to perform
operation = int(input(" Enter your choice of operation you want to perform: 1, 2, 3, 4 or 5 :\n"))
if operation == 1:
num_1 = int(input('Please, Enter the first number: \n'))
num_2 = int(input('Please, Enter the second number: \n'))
print('\nAddition of {} + {} = '.format(num_1, num_2), add(num_1, num_2),"\n")
elif operation == 2:
num_1 = int(input('Please, Enter the first number: \n'))
num_2 = int(input('Please, Enter the second number: \n'))
print('\nSubtraction of {} - {} = '.format(num_1, num_2), subtract(num_1, num_2),"\n")
elif operation == 3:
num_1 = int(input('Please, Enter the first number: \n'))
num_2 = int(input('Please, Enter the second number: \n'))
print('\nMultiplication of {} * {} = '.format(num_1, num_2), multiply(num_1, num_2),"\n")
elif operation == 4:
num_1 = int(input('Please, Enter the first number: \n'))
num_2 = int(input('Please, Enter the second number: \n'))
print('\nDivision of {} / {} = '.format(num_1, num_2), divide(num_1, num_2),"\n")
elif operation == 5:
print("Thank you for using calculator...")
break
else:
print("\nPlease enter Valid input\n")
Hi, This is the Basic console based calculator!
Please select which of the following arithmetic operation you want me to perform-
Add
Subtract
Multiply
Divide
Exit
Enter your choice of operation you want to perform: 1, 2, 3, 4 or 5 :
1
Please, Enter the first number:
10
Please, Enter the second number:
20
Addition of 10 + 20 = 30
Please select which of the following arithmetic operation you want me to perform-
Add
Subtract
Multiply
Divide
Exit
Enter your choice of operation you want to perform: 1, 2, 3, 4 or 5 :
2
Please, Enter the first number:
10
Please, Enter the second number:
20
Subtraction of 10 - 20 = -10
Please select which of the following arithmetic operation you want me to perform-
Add
Subtract
Multiply
Divide
Exit
Enter your choice of operation you want to perform: 1, 2, 3, 4 or 5 :
3
Please, Enter the first number:
10
Please, Enter the second number:
20
Multiplication of 10 * 20 = 200
Please select which of the following arithmetic operation you want me to perform-
Add
Subtract
Multiply
Divide
Exit
Enter your choice of operation you want to perform: 1, 2, 3, 4 or 5 :
4
Please, Enter the first number:
10
Please, Enter the second number:
20
Division of 10 / 20 = 0.5
Please select which of the following arithmetic operation you want me to perform-
Add
Subtract
Multiply
Divide
Exit
Enter your choice of operation you want to perform: 1, 2, 3, 4 or 5 :
5
Thank you for using calculator...
Enter the two integers that represent your math issue after choosing an operation. To obtain the solution to 10 x 20, for instance, enter 10 as your first number (num_1) and 20 as your second number (num_2).
When all requirements are satisfied for a particular operation—in this example, multiplication—the body of the else
statement is carried out, producing the result 200.
As illustrated below, these operations are also compatible with negative numbers.
Hi, This is the Basic console based calculator!
Please select which of the following arithmetic operation you want me to perform-
Add
Subtract
Multiply
Divide
Exit
Enter your choice of operation you want to perform: 1, 2, 3, 4 or 5 :
1
Please, Enter the first number:
-10
Please, Enter the second number:
20
Addition of -10 + 20 = 10
Please select which of the following arithmetic operation you want me to perform-
Add
Subtract
Multiply
Divide
Exit
Enter your choice of operation you want to perform: 1, 2, 3, 4 or 5 :
2
Please, Enter the first number:
-10
Please, Enter the second number:
-20
Subtraction of -10 - -20 = 10
Please select which of the following arithmetic operation you want me to perform-
Add
Subtract
Multiply
Divide
Exit
Enter your choice of operation you want to perform: 1, 2, 3, 4 or 5 :
3
Please, Enter the first number:
-10
Please, Enter the second number:
-20
Multiplication of -10 * -20 = 200
Please select which of the following arithmetic operation you want me to perform-
Add
Subtract
Multiply
Divide
Exit
Enter your choice of operation you want to perform: 1, 2, 3, 4 or 5 :
4
Please, Enter the first number:
-10
Please, Enter the second number:
20
Division of -10 / 20 = -0.5
Please select which of the following arithmetic operation you want me to perform-
Add
Subtract
Multiply
Divide
Exit
Enter your choice of operation you want to perform: 1, 2, 3, 4 or 5 :
5
Thank you for using calculator...
Additionally, the else block will be executed if the user selects an option that is not accessible in the range of 1 to 5, as illustrated below.:
Hi, This is the Basic console based calculator!
Please select which of the following arithmetic operation you want me to perform-
Add
Subtract
Multiply
Divide
Exit
Enter your choice of operation you want to perform: 1, 2, 3, 4 or 5 :
6
Please enter Valid input
Please select which of the following arithmetic operation you want me to perform-
Add
Subtract
Multiply
Divide
Exit
Enter your choice of operation you want to perform: 1, 2, 3, 4 or 5
As we used a while
True loop for the execution, the loop will then start over and ask the user for input. This will cause the program to run constantly until the user ends it by selecting the 5 exit option, where we used a break statement to exit the loop.
Whole numbers are used in this equation (known as integers). We are not using decimal points yet. The code’s int()
method is responsible for this. It may be modified by using the float()
method to allow for decimals, as illustrated below:
# Function to perform the arithmetic operation: Addition
def add(num_1, num_2):
return num_1 + num_2
# Function to perform the arithmetic operation: Subtraction
def subtract(num_1, num_2):
return num_1 - num_2
# Function to perform the arithmetic operation: Multiplication
def multiply(num_1, num_2):
return num_1 * num_2
# Function to perform the arithmetic operation: Division
def divide(num_1, num_2):
return num_1 / num_2
print("Hi, This is the Basic console based calculator!\n")
while True:
print("Please select which of the following arithmetic operation you want me to perform-\n\n" \
"1. Add\n" \
"2. Subtract\n" \
"3. Multiply\n" \
"4. Divide\n" \
"5. Exit\n")
# Taking the input from the user for which arithmetic operation to perform
operation = int(input(" Enter your choice of operation you want to perform: 1, 2, 3, 4 or 5 :\n"))
if operation == 1:
num_1 = float(input('Please, Enter the first number: \n'))
num_2 = float(input('Please, Enter the second number: \n'))
print('\nAddition of {} + {} = '.format(num_1, num_2), add(num_1, num_2),"\n")
elif operation == 2:
num_1 = float(input('Please, Enter the first number: \n'))
num_2 = float(input('Please, Enter the second number: \n'))
print('\nSubtraction of {} - {} = '.format(num_1, num_2), subtract(num_1, num_2),"\n")
elif operation == 3:
num_1 = float(input('Please, Enter the first number: \n'))
num_2 = float(input('Please, Enter the second number: \n'))
print('\nMultiplication of {} * {} = '.format(num_1, num_2), multiply(num_1, num_2),"\n")
elif operation == 4:
num_1 = float(input('Please, Enter the first number: \n'))
num_2 = float(input('Please, Enter the second number: \n'))
print('\nDivision of {} / {} = '.format(num_1, num_2), divide(num_1, num_2),"\n")
elif operation == 5:
print("Thank you for using calculator...")
break
else:
print("\nPlease enter Valid input\n")
Hi, This is the Basic console based calculator!
Please select which of the following arithmetic operation you want me to perform-
Add
Subtract
Multiply
Divide
Exit
Enter your choice of operation you want to perform: 1, 2, 3, 4 or 5 :
1
Please, Enter the first number:
5.5
Please, Enter the second number:
2.8
Addition of 5.5 + 2.8 = 8.3
Please select which of the following arithmetic operation you want me to perform-
Add
Subtract
Multiply
Divide
Exit
Enter your choice of operation you want to perform: 1, 2, 3, 4 or 5 :
2
Please, Enter the first number:
5.5
Please, Enter the second number:
2.8
Subtraction of 5.5 - 2.8 = 2.7
Please select which of the following arithmetic operation you want me to perform-
Add
Subtract
Multiply
Divide
Exit
Enter your choice of operation you want to perform: 1, 2, 3, 4 or 5 :
3
Please, Enter the first number:
5.5
Please, Enter the second number:
2.8
Multiplication of 5.5 * 2.8 = 15.399999999999999
Please select which of the following arithmetic operation you want me to perform-
Add
Subtract
Multiply
Divide
Exit
Enter your choice of operation you want to perform: 1, 2, 3, 4 or 5 :
4
Please, Enter the first number:
5.5
Please, Enter the second number:
2.8
Division of 5.5 / 2.8 = 1.9642857142857144
Please select which of the following arithmetic operation you want me to perform-
Add
Subtract
Multiply
Divide
Exit
Enter your choice of operation you want to perform: 1, 2, 3, 4 or 5 :
5
Thank you for using calculator...

Conclusion
We wrote a Python calculator program based on the user’s input of numbers and operators, just like a real calculator.
This is only one simple application that we have developed; with Python programming, we can make many more.
Python is one of the popular and versatile programming languages. It is beginner friendly and therefore the best programming language to start with. It is considered to be one of the most used languages in the world.
Python is widely used in various fields such as data analysis, machine learning, software testing and prototyping, web development, and in many other areas.
We provide 1:1 live interactive online coding classes for kids with expert coding instructors. Along with lifetime access to course content and downloadable learning resources, we also cater to every child’s need by providing a personalized journey. Python for kids will help your child to enhance cognitive, logical, and computational skills.